Java EnumMap
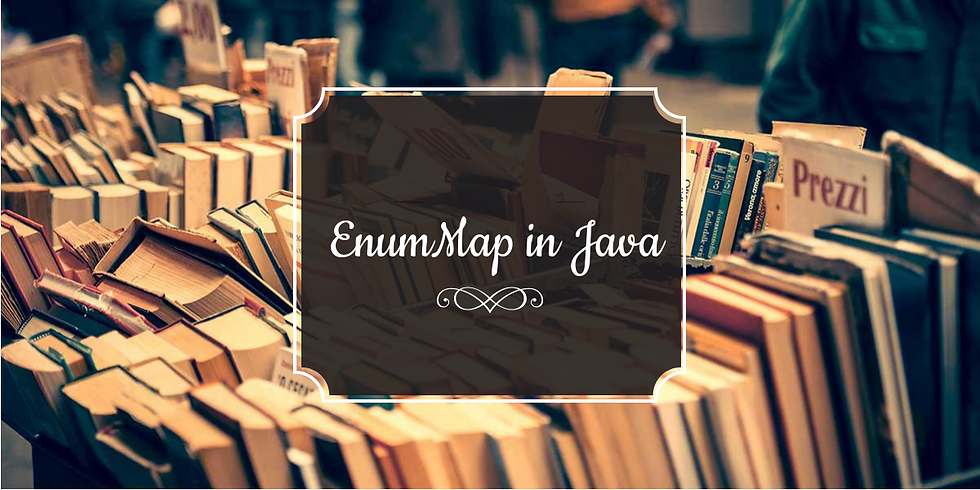
What is EnumMap in Java?
Java EnumMap class Declaration
Features:
Java EnumMap inherits AbstractMap class.
All keys of each EnumMap instance must be keys of a single enum type.
Java EnumMap does not permits null key, but permits multiple null values.
Enum maps are maintained in the natural order of their keys as the order in which the enum constants are declared).
Iterators returned by the collection views are weakly consistent: they will never throw ConcurrentModificationException and they may or may not show the effects of any modifications to the map that occur while the iteration is in progress.
Java EnumMap is not synchronized.
Java EnumMap is not thread-safe. You can make thread-safe by doing Collections.synchronizedMap(new EnumMap<EnumKey, V>(...));
Java EnumMap implementation provides constant-time performance for the basic operations (like get, and put)
Java EnumMap performance is better than HashMap.
Let’s create a small example so that we can analyze it more
Create an ENUM:
package com.java.gyan.mantra.poc;
public enum Activity {
APPROVED, DENIED, CANCELLED
}
Create one class where we can do some CRUD operation with EnumMap
package com.java.gyan.mantra.poc;
import java.util.EnumMap;
import java.util.Map;
public class EnumMapDemo {
public static void main(String[] args) {
Map<Activity, String> enumMap = new EnumMap<>(Activity.class);
enumMap.put(Activity.APPROVED, "Approved");
enumMap.put(Activity.DENIED, "Denied");
enumMap.put(Activity.CANCELLED, "Cancelled");
System.out.println("All key-value in enum map : " + enumMap);
System.out
.println("Getting value for key = " + Activity.CANCELLED + " is : " + enumMap.get(Activity.CANCELLED));
// checking if EnumMap contains a particular key
System.out.println("Does enum map has :" + Activity.DENIED + " " + enumMap.containsKey(Activity.DENIED));
// checking if EnumMap contains a particular value
System.out.println("Does enum map has Cancelled value: " + enumMap.containsValue("Cancelled"));
System.out.println("Removing key = " + Activity.APPROVED + " from enum map and value is : "
+ enumMap.remove(Activity.APPROVED));
}
}
Output:
All key-value in enum map: {APPROVED=Approved, DENIED=Denied, CANCELLED=Cancelled}
Getting value for key = CANCELLED is: Cancelled
Does enum map has: DENIED true
Does enum map has cancelled value: true
Removing key = APPROVED from enum map and value is: Approved
Let’s see the approaches to iterate an EnumMap:
package com.java.gyan.mantra.poc;
import java.util.EnumMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public class EnumMapDemo {
public static void main(String[] args) {
Map<Activity, String> enumMap = new EnumMap<>(Activity.class);
enumMap.put(Activity.APPROVED, "Approved");
enumMap.put(Activity.DENIED, "Denied");
enumMap.put(Activity.CANCELLED, "Cancelled");
// iterate EnumMap using forEach
for (Activity key : enumMap.keySet()) {
System.out.println(key + ":" + enumMap.get(key));
}
// iterate EnumMap using Iterator
Set<Entry<Activity, String>> set = enumMap.entrySet();
Iterator<Entry<Activity, String>> itr = set.iterator();
while (itr.hasNext()) {
Map.Entry<Activity, String> map = itr.next();
System.out.println(map.getKey() + ":" + map.getValue());
}
// iterate EnumMap using java 8 for each and lambda expression
enumMap.forEach((k, v) -> {
System.out.println(k + " :" + v);
});
}
}
Now let’s discuss Difference between EnumMap and HashMap in Java
EnumMap vs HashMap in Java
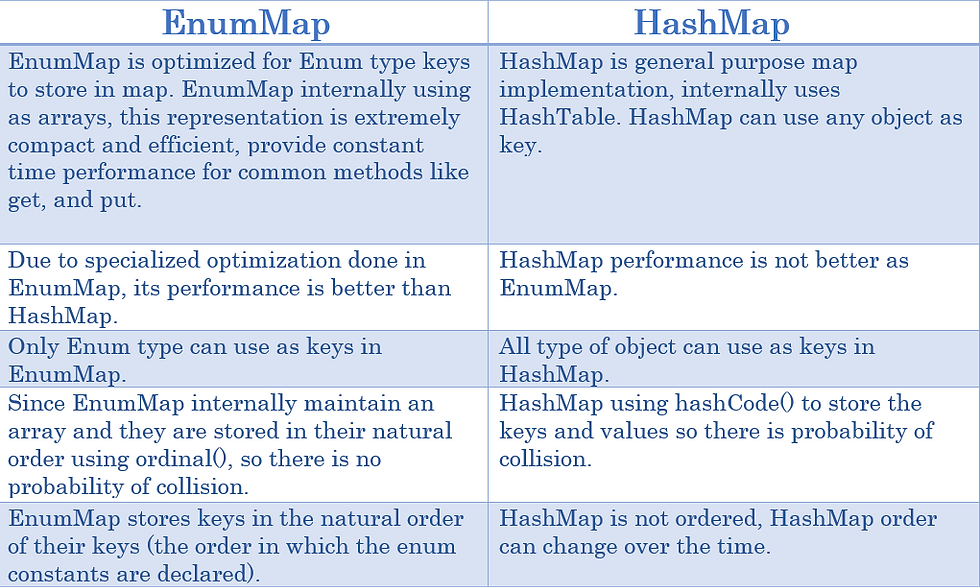
Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above or having any doubt?
If you liked this article, please leave comment, subscribe it here